Securing data through encryption and decryption holds significant importance within the realm of web development. This is particularly pertinent when managing confidential information such as passwords, API keys, or personal data. In the following comprehensive tutorial, we shall lead you through the intricate journey of encoding and decoding strings in PHP, guaranteeing the utmost security for your valuable data. Let’s commence our exploration!
The Significance of Encrypting and Decrypting Strings in PHP
In the digital age, safeguarding sensitive information is paramount. Whether you’re managing user credentials, protecting your database, or securing confidential data, encryption serves as your digital fortress. By converting plain text into a cryptic code and subsequently decrypting it when needed, you erect an impenetrable barrier against prying eyes. Let’s delve deeper into why encrypting and decrypting strings in PHP is essential and explore the myriad of benefits it offers:
Why Encrypt and Decrypt Strings in PHP?
- Protection of Sensitive Information: Encryption acts as a robust shield, rendering your data indecipherable to unauthorized individuals. Even if malevolent actors breach your system, the information remains inscrutable without the requisite decryption key;
- Regulatory Compliance: Many industries are bound by strict data protection regulations. Encrypting sensitive data ensures compliance with these regulations, sparing you from potential legal and financial ramifications;
- Secure Communication: When transmitting sensitive data across networks or between systems, encryption ensures that the information remains secure during transit, thwarting eavesdroppers;
- Trust Building: Demonstrating a commitment to data security by encrypting user information can foster trust and confidence among your users or clients;
- Prevention of Insider Threats: Encryption acts as a safeguard against internal threats, preventing employees or insiders with malicious intent from accessing sensitive data.
Navigating PHP’s Encryption Algorithm Landscape
PHP offers a versatile array of encryption algorithms, each tailored to specific security needs and scenarios. Choosing the right algorithm is akin to selecting the right tool for the job. Here’s an exploration of some commonly used encryption algorithms in PHP and when to employ them:
Choosing the Right Encryption Algorithm
- AES (Advanced Encryption Standard):
- Symmetric Encryption: AES is a go-to choice when you need both the encryption and decryption processes to use the same key. It’s highly efficient for securing data at rest;
- Recommended Usage: Protecting sensitive files, encrypting data stored in databases, securing communication within a closed system.
- RSA (Rivest-Shamir-Adleman):
- Asymmetric Encryption: RSA employs a pair of keys, one for encryption and another for decryption. It’s ideal for secure communication and digital signatures;
- Recommended Usage: Securing communication over the internet, ensuring data authenticity, and establishing secure channels between different entities;
- bcrypt;
- Password Hashing: While not strictly an encryption algorithm, bcrypt is invaluable for securely storing and verifying passwords. It adds a layer of security by hashing passwords and salting them;
- Recommended Usage: Storing and verifying user passwords, enhancing security against password cracking attempts.
Creating an index.php File for Encrypting and Decrypting Strings in PHP
In the world of web development, security is paramount. One of the essential aspects of web security is the ability to encrypt and decrypt sensitive information, such as passwords, user data, or confidential messages. In this tutorial, we’ll walk you through the process of creating an index.php file that will allow you to encrypt and decrypt strings in PHP using the AES-256-CBC encryption method. We’ll also provide recommendations for enhancing security and best practices for handling encryption keys.
Step 1: Setting Up the HTML Structure
To get started, create an index.php file and set up the basic HTML structure. You can use any code editor or IDE of your choice. In this example, we’ve added a title and some minimal CSS styling for better presentation.
<!DOCTYPE html>
<html>
<head>
<title>Demo Encrypt Decrypt String PHP - AllPHPTricks.com</title>
<style>
body {
font-family: Arial, sans-serif;
line-height: 1.6;
}
</style>
</head>
<body>
<div style="width:700px; margin:50 auto;">
<h1>Demo Encrypt Decrypt String PHP</h1>
<!-- PHP code will go here -->
</div>
</body>
</html>
Step 2: Adding PHP Code for Encryption and Decryption
Now, let’s add the PHP code for encrypting and decrypting strings. We’ve encapsulated the encryption and decryption functionality within a encrypt_decrypt function. Here’s the PHP code:
<?php
// Function to Encrypt Decrypt String
function encrypt_decrypt($action, $string) {
$output = false;
$encrypt_method = "AES-256-CBC";
// Update your secret key before use
$secret_key = '$7PHKqGt$yRlPjyt89rds4ioSDsglpk/';
// Update your secret iv before use
$secret_iv = '$QG8$hj7TRE2allPHPlBbrthUtoiu23bKJYi/';
// hash
$key = hash('sha256', $secret_key);
// iv - encrypt method AES-256-CBC expects 16 bytes - else you will get a warning
$iv = substr(hash('sha256', $secret_iv), 0, 16);
if ( $action == 'encrypt' ) {
$output = openssl_encrypt($string, $encrypt_method, $key, 0, $iv);
$output = base64_encode($output);
} else if( $action == 'decrypt' ) {
$output = openssl_decrypt(base64_decode($string), $encrypt_method, $key, 0, $iv);
}
return $output;
}
// Plain String
$string = "AllPHPTricks";
echo "<p><strong>Plain String:</strong> ". $string . "</p>";
// Encrypted String
$encrypted_string = encrypt_decrypt('encrypt', $string);
echo "<p><strong>Encrypted String:</strong> ". $encrypted_string . "</p>";
// Decrypted String
$decrypted_string = encrypt_decrypt('decrypt', $encrypted_string);
echo "<p><strong>Decrypted String:</strong> ". $decrypted_string . "</p>";
?>
Step 3: Key Management and Best Practices
Now that you have a basic understanding of encrypting and decrypting strings in PHP, it’s crucial to follow best practices for key management and security:
- Key Generation: Generate strong, unique encryption keys. Avoid using hardcoded keys in your code;
- Key Storage: Store encryption keys securely, separate from the codebase;
- Key Rotation: Regularly rotate encryption keys to enhance security;
- Secure Transport: Ensure secure transmission of encrypted data over networks (e.g., HTTPS);
- Error Handling: Implement robust error handling to deal with encryption/decryption failures;
- Logging: Log encryption-related events for auditing and debugging purposes;
- Testing: Thoroughly test your encryption and decryption processes.
Read about the Power of PHP: Seamlessly import CSV files into MySQL databases with this step-by-step guide for efficient data management.
Enhancing Security through Effective Key Management
Implementing robust security measures is essential for safeguarding sensitive data, especially when it comes to the management of encryption keys. The following strategies can significantly enhance the security of encryption keys:
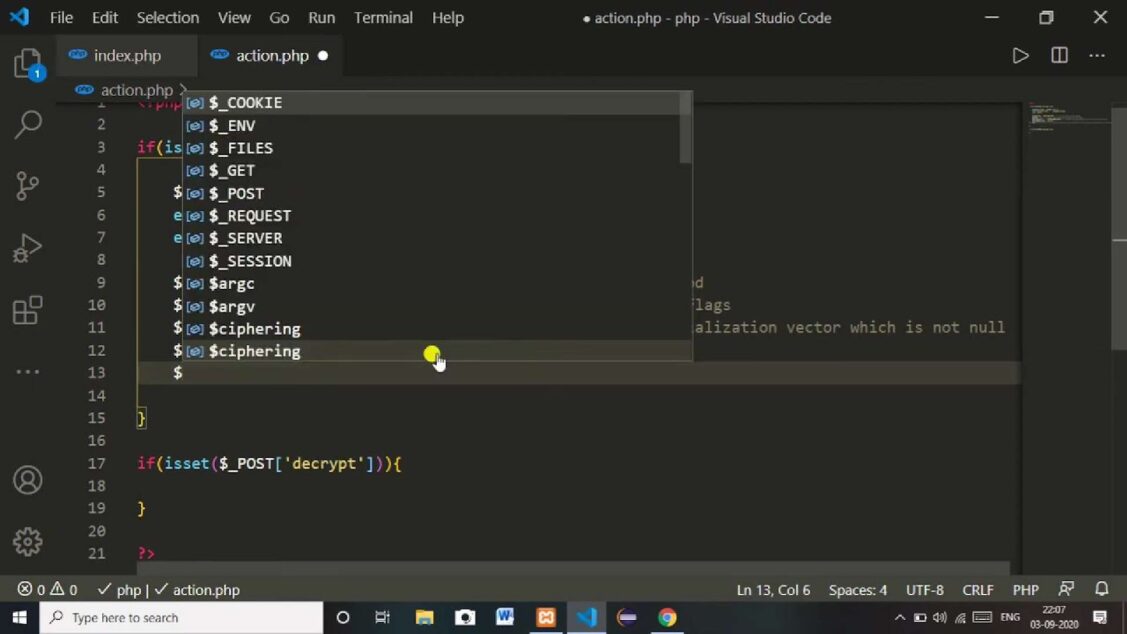
- Utilize Secure Storage Solutions: Avoid embedding encryption keys directly in your application’s source code or storing them in unencrypted files. These practices are vulnerable to security breaches;
- Employ Environment Variables: A safer approach is to store encryption keys as environment variables. This method keeps them outside the codebase and reduces the risk of accidental exposure;
- Leverage Key Management Services: Consider using specialized key management systems like AWS Key Management Service (KMS) or HashiCorp Vault. These services offer robust security features, including key rotation, access control, and audit trails, thus providing an additional layer of security.
Advanced String Encryption Techniques in PHP
Ensuring the security of data through encryption in PHP applications involves several best practices:
- Regularly Update PHP: Stay current with the latest PHP versions. Each update often includes improvements in security, which can significantly reduce vulnerabilities;
- Use Strong and Unique Keys: Always opt for strong, unique encryption keys for each task. Weak or reused keys can be easily compromised, putting your data at risk;
- Implement Error Handling: Proper error handling is crucial. It helps in managing exceptions that occur during encryption and decryption processes, ensuring the stability and security of your application;
- Consider Established Libraries: For applications requiring advanced security measures, integrating a well-established encryption library can be beneficial. These libraries are often rigorously tested and updated, offering reliable and secure encryption solutions.
Conclusion
Mastering the art of encrypting and decrypting strings in PHP stands as a crucial proficiency when it comes to fortifying the confidentiality of sensitive data within your web applications. Your ability to safeguard valuable information hinges on your adeptness in selecting the appropriate encryption algorithm, upholding the utmost security in key management, and adhering diligently to best practices. Through these measures, you can guarantee that your data remains impervious to prying eyes and unauthorized access.
Integrating robust encryption methodologies into your PHP applications represents an elemental stride toward preserving the sanctity of your users’ data and the overall integrity of your system. By faithfully embracing the directives delineated in this comprehensive manual, you can embark on the journey of encrypting and decrypting strings in PHP with unwavering confidence.
Keep an eye out for forthcoming pearls of wisdom regarding PHP security and web development in our future publications!